Introduction
In this blog post, we will explore vital topics that form the bedrock of multithreaded programming. We will understand the fundamental concepts of thread creation to delving into advanced techniques like synchronization and inter-thread communication, this blog post aims to make you understand the knowledge and tools which is necessary to master the art of multithreading.
We will thoroughly understand the concept of thread priority & explore methods to prevent stop execution using diffrent methods & how to handle deadlock situations. Moreever, we will learn the concept of daemon threads and their significance in the Java ecosystem & many more other topics as well.
What is Multitasking in Java
Executing multiple tasks simultaneously is known as multitasking. To illustrate, consider the analogy of a person driving a car while also listening and handling a phone call. In this scenario, the individual executing several tasks simultaneously which shows =as an example of multitasking.
Type of Multitasking
1: Process Based Multitasking
Executing several task simultaneously where each task is a separate independent process is called as Process Based Multiasking. For example, we can listen music along with writing code on code editor while downloading the file in our computer system. Process Based Multitasking is best suiable for OS(Operating System ) level.
1: Thread Based Multitasking
Executing multiple task simultaneously where each task is a separate independent part of the same program is called as Thread Based Multiasking. Each indepandent part is called as Thread. Thread Based Multiasking is best suitable at programatic level.
Advantage of Multitasking
Whether it’s process-based multitasking or thread-based multitasking, the primary goal is to reduce system response time and enhance performance. To develop web server and application server we use multithreading where multiple request handled simultaneously. So, whenever independent jobs are required to execute a the same time, the best suitable approch is using multithreading. When compared with old languages, developeing multithreaded application in Java is very easy because Java provides inbuild support for multithreading with rich API’s(Thread, Runnable, Threadgroup etc).
Mulithreading
Wha is Thread?
Thread is a separate flow of execution which are considered as ightweight subprocesses that share the same memory space. For every thread there is a separate indepandent job to perform. Multithreading in Java is a feature that allows multiple threads to run simultaneously within a single program . It improves the performance and responsiveness of the application.
Wha is Thread?
Thread is a separate flow of execution which are considered as ightweight subprocesses that share the same memory space. For every thread there is a separate indepandent job to perform. Multithreading in Java is a feature that allows multiple threads to run simultaneously within a single program . It improves the performance and responsiveness of the application.
Define a Thread
We can define the thread in the following 2 ways
1: By Extending Thread Class
2: By Implementing Runnable Interface
By Extending Thread Class
public class Test {
public static void main(String[] args) {
MyThread t1 = new MyThread();
t1.start();
for (int i = 1; i <= 5; i++) {
System.out.println("Main Thread " + i);
}
}
}
class MyThread extends Thread {
//Job of the thread 👇
@Override
public void run() {
// TODO Auto-generated method stub
for (int i = 1; i <= 5; i++) {
System.out.println("Hello " + i);
}
}
}
Thread Scheduler
Thread scheduler is the part of the JVM and responsible to schedule the threads. If multiple threads are waiting to get the chance of execution then in which order threads will be executed is decided by thread scheduler.
We can not expect exact algorithm followed by thread scheduler , it varied from JVM to JVM. Hence, we can not expect thred execution order and exact output. Hence, whenver situation comes to multithreading there is no gurentee for the exact output but we can provide several possible outputs .
As shown in the above example, everytime we get diffrent output & no oine can gurentee about the output.
Importance of Thread classs start() method:
It is responsible to register the thread with thread scheduler & all other mandatory activities. Hence without executing thread class start merhod. There is no chance of starting a new thread in java. Due to this thread class start method is considered as heart of multithreading.
Job of Start() method is:-
1 : Register this thread with Threadscheduler
2: Perform other activities
3: Invoke run() method
if we are not overriding run() method then Thread class run() mehod executed having empty implementation. Hence, we will not get any output.
What happend if we override start() method ?
If we override start() mehod then our start() method will be executed just like a normal method call & new thread would not created. Here, run() method will not get the chance to execute. So it is necessary to not override start() method in our thread class.
public class Test {
public static void main(String[] args) {
MyThread t1 = new MyThread();
t1.start();
for (int i = 1; i <= 5; i++) {
System.out.println("Main Thread " + i);
}
}
}
class MyThread extends Thread {
@Override
public void start() {
// TODO Auto-generated method stub
System.out.println("Override Start method call");
}
@Override
public void run() {
// TODO Auto-generated method stub
for (int i = 1; i <= 5; i++) {
System.out.println("Hello " + i);
}
}
}
Output:
Override Start method call
Main Thread 1
Main Thread 2
Main Thread 3
Main Thread 4
Main Thread 5
What is Thread Priority?
Every thread in java has some priority ,it may be default priority generated by JVM or customize priority provided by programmer. The valid range of thread priority is 1 to 10 where 1 is Min Priority and 10 is Max Priority.
Thread class defines the following constants to represent some statndards priorities.
Thread.MIN_PRIORITY—>1
Thread.NORM_PRIORITY—>5
Thread.MAX_PRIORITY—->10
Thread scheduler will use priorities while allocating processor. The thread which is having highest priority will get the chance first.
If two threads having same priority then we cant expect exact execution order. It depends on Thread Scheduler.
Thread class define the following method to set and get the priority of a thread.
public final int getPriority();
public final void setPriority(ing p);
Allowed values range is 1 to 10 otherwise there will be a run time exception IllegalArgumentException caught.
Default Priority
The default priority only for main thread is 5 but all remaining threads default priority will be inherited from parent to child that is whatever priority parent thread has , the same priority will be there for the child thread.
public class RunnableTest {
public static void main(String[] args) {
System.out.println("Current Thread Priority:"+Thread.currentThread().getPriority());
// Change Curren Thread Priority
Thread.currentThread().setPriority(7);
System.out.println("Now Current Thread Priority is:"+Thread.currentThread().getPriority());
Thread t=new Thread();
System.out.println( "Child Thread Priority: "+t.getPriority());
}
Output:
Current Thread Priority:5
Now Current Thread Priority is:7
Child Thread Priority: 7
Note: Some plateform dont provide support for Thread Priority.
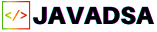