
Side-effect in Javascript
In programming, particularly in JavaScript, a “side effect” refers to any observable change that a function or expression makes outside its own scope. These changes can be modifications to the program state, variables, or interactions with the external world, such as modifying global variables, logging to the console, writing to a file, making network requests, or updating the user interface.
Functions that have side effects are sometimes called “impure functions” because their output is not solely determined by their input, but also by the external state they interact with. In contrast, “pure functions” are functions that produce the same output for the same input and have no side effects.
Managing side effects is important because they can lead to unintended behavior and make code harder to reason about and test. Pure functions, on the other hand, are easier to understand, test, and maintain since they don’t rely on hidden dependencies or change the program’s state unexpectedly.
To reduce side effects and make code more maintainable and predictable, developers often use techniques like functional programming, immutability, and separation of concerns. Libraries like Redux in JavaScript are designed to help manage state and side effects in a more controlled manner.
Here are a few examples of side effects in JavaScript:
1:Modifying a global variable:
In this example, the incrementCounter
function has a side effect because it modifies the global variable counter
outside of its own scope.
2: Console logging:
The greet
function has a side effect because it logs a message to the console, which is an external interaction outside of the function’s core functionality.
3:DOM manipulation:
In this example, when the button is clicked, the event listener function changes the text of the button. This is a side effect because it modifies the DOM, which is outside of the function’s scope.
4:Making HTTP requests
The fetchData
function has a side effect because it makes an HTTP request to an external API and logs the retrieved data to the console.
These examples demonstrate how side effects can occur in JavaScript when functions or expressions interact with the external environment, leading to changes that go beyond their immediate scope. Managing and minimizing such side effects is crucial for writing maintainable and predictable code.
👉 Minimizing side effects is essential for writing maintainable and predictable code. To do so, developers can follow these practices:
- Use Pure Functions: Pure functions are functions that have no side effects and always produce the same output for the same input. They rely solely on their arguments and don’t modify any external state.
- Avoid Modifying Global State: Instead of modifying global variables directly, it’s better to encapsulate state within functions and pass data through function arguments and return values.
- Immutability: Prefer using immutable data structures when possible. Immutable data ensures that data remains constant and cannot be changed once created, which helps in reducing side effects.
- Separate Side Effects from Core Logic: Keep side-effectful code separate from the core logic. For example, separate functions for data manipulation and functions for I/O operations.
- Use Functional Programming Principles: Embrace functional programming concepts, like higher-order functions and map/filter/reduce, to handle data transformations without side effects.
Minimizing side effects leads to code that is easier to understand, test, and maintain. It promotes better predictability, enhances debugging, and reduces unintended consequences caused by hidden dependencies. Additionally, code with fewer side effects is less prone to unexpected bugs and easier to optimize for performance.
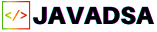