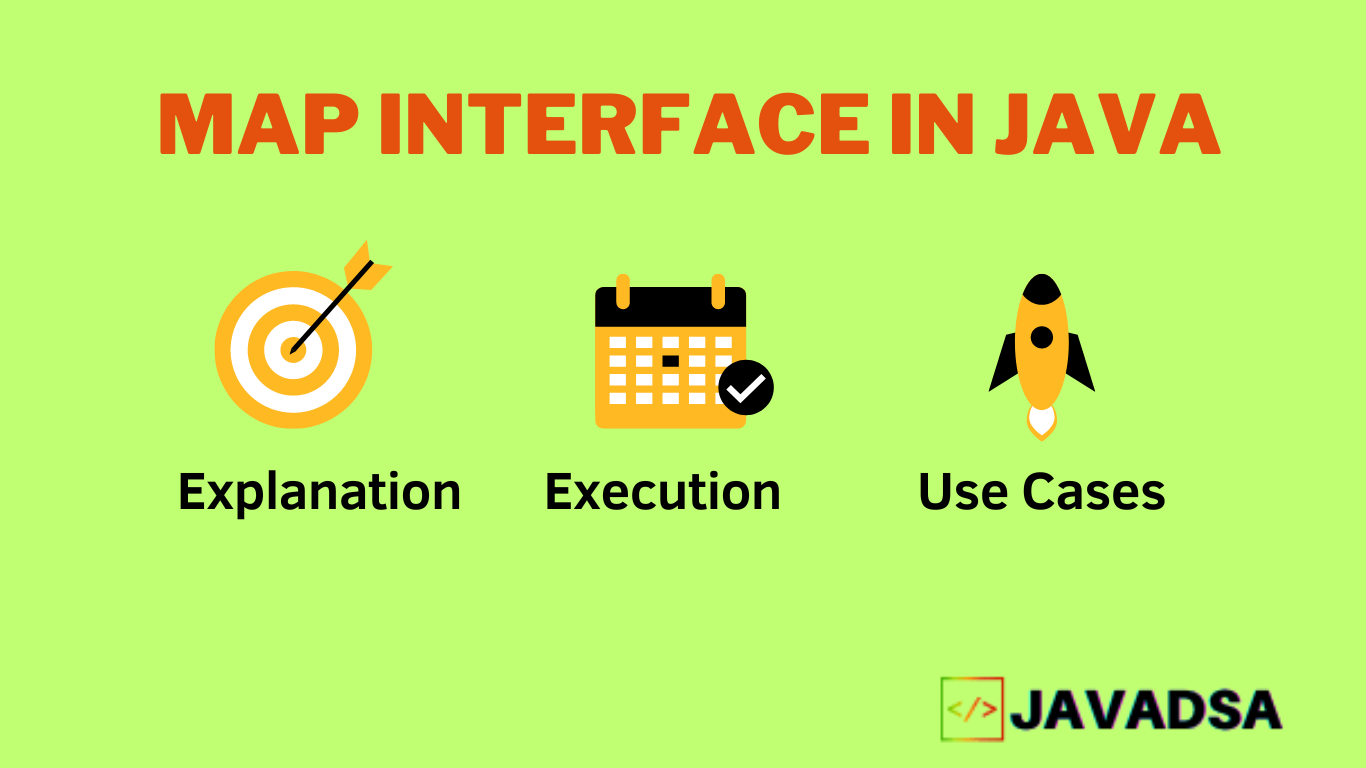
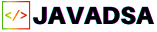
Inroduction In any programming languages, efficiency and readability are considered a crucial aspect. Java...
Table of Contents What is Functional Interface ? In Java, functional Interface is used ...
A Lambda Expression in Java is a feature introduced in Java 8. It helps...
Agenda for this blog is to explore Java 8 features which was a big...