
Hibernate gives us two ways to get data from the database using their primary key. These are called get() and load() methods. Both methods are used to achieve the same goal, which is getting data from the database. We often use both methods without knowing exactly how they are different. Let’s take a closer look at them and understand their main distinctions, as well as how they work behind the scenes.
Two get the clear idea, Let’s check one by one using code example.
Common things about get() & load() method
1: Both methods are used to get an object from the database based given primary key.
2: Both methods belongs to session interface & call by session.get & session.load()
Here we have a Entity class named Employee.java
package com.hiber.getloadmethod;
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class Employee {
@Id
int id;
String empName;
String designation;
public Employee() {
super();
// TODO Auto-generated constructor stub
}
public Employee(int id, String empName, String designation) {
super();
this.id = id;
this.empName = empName;
this.designation = designation;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getEmpName() {
return empName;
}
public void setEmpName(String empName) {
this.empName = empName;
}
public String getDesignation() {
return designation;
}
public void setDesignation(String designation) {
this.designation = designation;
}
@Override
public String toString() {
return "Employee [id=" + id + ", empName=" + empName + ", designation=" + designation + "]";
}
}
mysql> select * from employee;
+----+------------------------+-----------+
| id | designation | empName |
+----+------------------------+-----------+
| 1 | Database Administrator | Sachindra |
| 2 | Software Engineer | Sanjeev |
+----+------------------------+-----------+
As we can see we have 2 objects which is already saved in the database.
Lets see the diffrences between get() & load() methods
1: session.load()
When session.load() method called, hibernate prepare a fake objects based on the given identifier in the memory. As we can see above we have a Entity class Employee having properties id,name & department.
Here id is a primary key as we have annotate it @Id in the entity class.
If we hit session.load(Employee.class, 1) , hibernate will create a fake object in the memory with id 2 but rest of the properties of the employee class will not be even initialized. The actual data is loaded from the database only when you access any property of the proxy object This is the reason this method also referred as Lazy Loading .

If the object with the given primary key does not found then it throw an exception called ObjectNotFoundException
lets try to get an object whose primary key is 3 (which doesnt exist in database) then it will throw an exception i.e ObjectNotFoundException

1: session.get()
This get() method retrieve an object from the database. based on given PK(primary key). If the object is found based on given primary key then it provide the object & return it otherwise it returns null.

How to choose between get() & load() method. 🤔
We can choose above method based on our use case and might be difrent for diffrent people & its totaly depands upon the situation.

Here is the summry table

Download source code here ⬇️
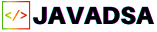