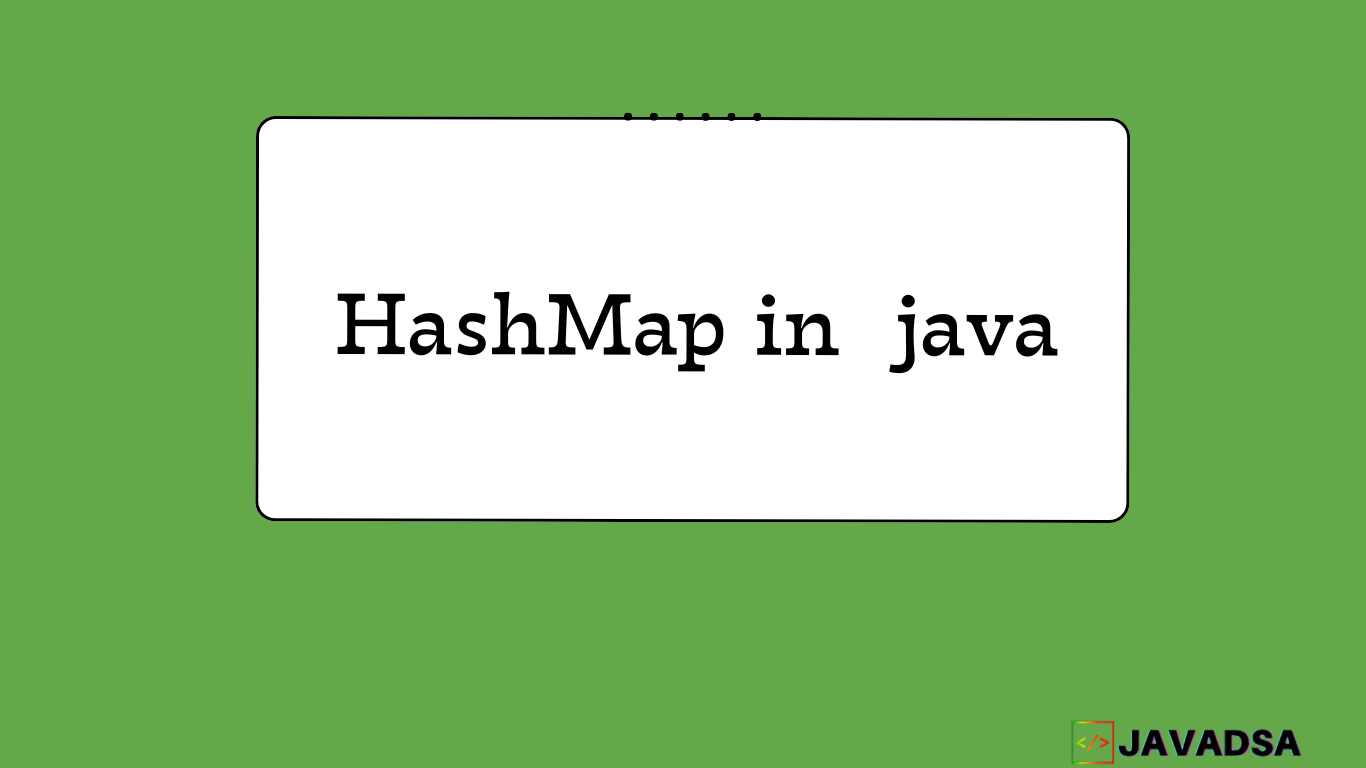
HashMap is a fundamental data structure in Java that provides an efficient way to store and retrieve key-value pairs. It’s widely used in various programming scenarios due to its quick access and lookup times. In this article, we’ll explore how HashMap works in Java and the underlying principles that make it a powerful tool for developers.
Understanding HashMap Basics
At its core, a HashMap is a collection that stores data in the form of key-value pairs. Each key is unique, and it’s used to access its associated value. When you insert a key-value pair into a HashMap, the key is hashed to generate an index that points to the location in memory where the value is stored.
Hashing and Indexing
Hashing is a crucial aspect of HashMaps. When you add a key-value pair to a HashMap, the key is passed through a hash function that produces a hash code—a unique numeric representation of the key. This hash code is used to determine the index in the internal array where the value will be stored.
Handling Collisions
Since multiple keys can produce the same hash code (a phenomenon known as a collision), HashMaps need a strategy to manage such situations. To address collisions, Java’s HashMap employs a technique called chaining. In this approach, each index in the internal array holds a linked list of key-value pairs that share the same hash code. This linked list ensures that all key-value pairs are stored and retrievable, even when they have colliding hash codes.
Performance and Time Complexity
HashMaps offer exceptional performance when it comes to retrieval and insertion. On average, these operations take constant time—O(1)—thanks to the hash-based indexing. However, in the worst case scenario, when there are many collisions leading to long linked lists, the performance can degrade to O(n), where n is the number of elements in the list. This is a reminder of the importance of choosing a good hash function and handling collisions effectively.
Load Factor and Resizing
As you add more elements to a HashMap, the ratio of filled buckets to the total number of buckets (known as the load factor) increases. When the load factor surpasses a certain threshold, the HashMap automatically resizes itself by creating a new, larger internal array. This process helps maintain efficient performance by reducing the chances of collisions and excessively long linked lists.
Conclusion
In summary, HashMaps in Java offer a powerful and efficient way to manage key-value pairs. By using hashing and indexing, they provide quick access and retrieval times, making them invaluable in various programming scenarios. Understanding how HashMaps work, including their hashing mechanism, collision handling, and resizing strategy, equips developers with the knowledge needed to make informed decisions when using this data structure. As you continue your journey in Java programming, delving deeper into HashMaps will undoubtedly enhance your ability to design efficient and optimized solutions.
Let’s walk through a simple example of how a HashMap works in Java. In this example, we’ll create a HashMap to store the names and ages of individuals.
import java.util.HashMap;
public class HashMapExample {
public static void main(String[] args) {
// Create a HashMap to store names and ages
HashMap<String, Integer> ageMap = new HashMap<>();
// Adding key-value pairs to the HashMap
ageMap.put("Alice", 25);
ageMap.put("Bob", 30);
ageMap.put("Carol", 28);
ageMap.put("David", 22);
// Accessing values using keys
System.out.println("Age of Alice: " + ageMap.get("Alice")); // Output: Age of Alice: 25
System.out.println("Age of Bob: " + ageMap.get("Bob")); // Output: Age of Bob: 30
// Updating a value
ageMap.put("Carol", 29); // Carol's age is updated to 29
// Checking if a key exists
System.out.println("Is Carol's age present? " + ageMap.containsKey("Carol")); // Output: Is Carol's age present? true
System.out.println("Is Eve's age present? " + ageMap.containsKey("Eve")); // Output: Is Eve's age present? false
// Removing a key-value pair
ageMap.remove("David"); // David's age is removed from the map
// Iterating through the HashMap
System.out.println("Names and Ages:");
for (String name : ageMap.keySet()) {
int age = ageMap.get(name);
System.out.println(name + ": " + age);
}
}
}
In this example, we import the HashMap
class from the java.util
package. We then create a HashMap called ageMap
with keys of type String
(names) and values of type Integer
(ages).
We use the put()
method to add key-value pairs to the HashMap. The keys are unique, and the HashMap ensures that there are no duplicate keys.
We retrieve values using the get()
method, passing in the key. We update values using the put()
method with an existing key. We can check if a key exists using the containsKey()
method.
To remove a key-value pair, we use the remove()
method, specifying the key.
Finally, we iterate through the HashMap using the keySet()
method, which returns a set of all keys. We use the get()
method to fetch the associated value for each key.
This example demonstrates the basic operations of adding, accessing, updating, and removing key-value pairs in a HashMap. Behind the scenes, the HashMap uses hash codes and buckets to efficiently manage the data, ensuring fast retrieval times even for a large number of elements.
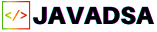